Latest posts
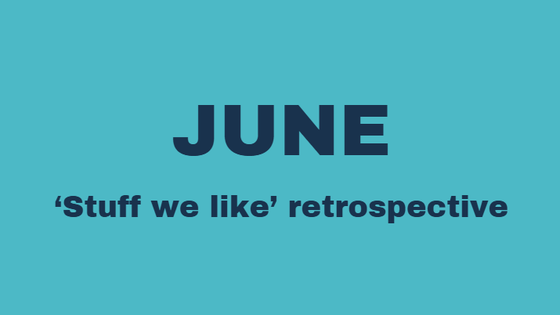
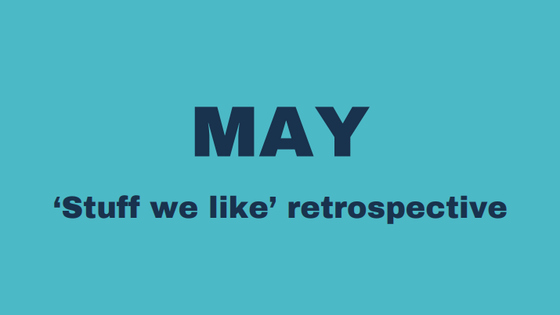
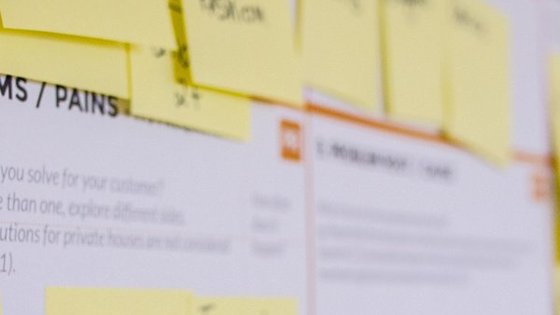
The VESPR lead qualification framework: is this project for us?
How does an agency choose who it works with? And which projects drag us down?
Read moreHow does an agency choose who it works with? And which projects drag us down?
Read more
We don't send many emails, but when we do you'll want to read them.
Make sure you're on the list.